MoreWindow.c File Reference
#include "MorePrefix.h"
#include "MoreWindow.h"
#include "MoreStringMgr.h"
#include "MorePrivate.h"
#include <PalmTypes.h>
#include <SystemMgr.h>
#include <Window.h>
Include dependency graph for MoreWindow.c:
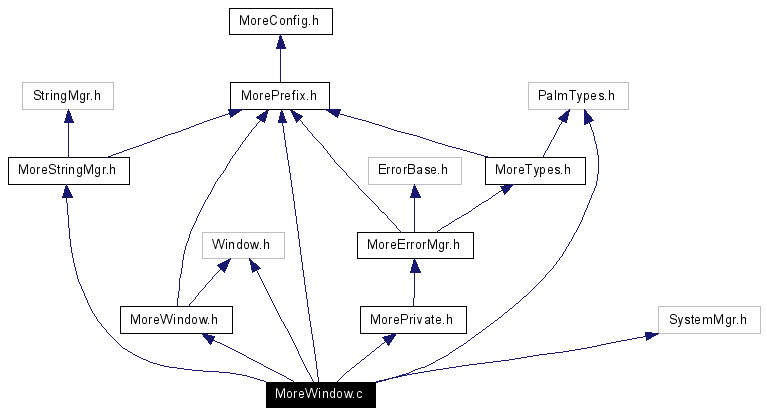
Go to the source code of this file.
Functions | |
Err | MWinScreenToDefault (MOREPALMOS_NOPARAMS) |
Switch screen back to default. | |
Err | MWinScreenToDepth (UInt8 depth) |
Switch screen to a particular depth. | |
Coord | MWinDrawAlignStr (const char *str, Coord x, Coord y, Alignment align) |
Draws a string at input coordinates using an alignment. | |
Coord | MWinDrawAlignChars (const char *str, UInt32 len, Coord x, Coord y, Alignment align) |
Draws text at input coordinates using an alignment. | |
Coord | MWinDrawAlignStrTrunc (const char *str, Coord x, Coord y, Coord availWidth, Alignment align) |
Draws a string at input coordinates using an alignment, truncated to a maximum width. | |
Coord | MWinDrawAlignCharsTrunc (const char *str, UInt32 len, Coord x, Coord y, Coord availWidth, Alignment align) |
Draws text at input coordinates using an alignment, truncated to a maximum width. |
Function Documentation
|
Draws text at input coordinates using an alignment. Draw text at x,y with alignment and return the new text position. Alignment determines which way the text is drawn. If you pass an alignment of Left:
If you pass an alignment of Right
If you pass an alignment of Center:
Definition at line 64 of file MoreWindow.c. 00066 { 00067 Coord strWidth; 00068 _require( str, noString ); 00069 _require( len, noString ); 00070 strWidth = FntCharsWidth( str, len ); 00071 if ( align != Left ) 00072 { 00073 x -= ( align == Center ) ? ( strWidth >> 1 ) : strWidth; 00074 } 00075 WinPaintChars( str, len, x, y ); 00076 x += strWidth; // return x+strWidth; generates larger code 00077 // fall thru 00078 noString: 00079 return x; 00080 }
|
|
Draws text at input coordinates using an alignment, truncated to a maximum width. Draw text at x,y with alignment and return the new text position, using a maximum of availWidth pixels. Alignment determines which way the text is drawn. If you pass an alignment of Left:
If you pass an alignment of Right
If you pass an alignment of Center:
Definition at line 91 of file MoreWindow.c. 00093 { 00094 Coord strWidth; 00095 _require( str, noString ); 00096 _require( len, noString ); 00097 strWidth = FntCharsWidth( str, len ); 00098 if ( strWidth > availWidth ) 00099 strWidth = availWidth; 00100 if ( align != Left ) 00101 { 00102 x -= ( align == Center ) ? ( strWidth >> 1 ) : strWidth; 00103 } 00104 WinDrawTruncChars( str, len, x, y, availWidth ); 00105 x += strWidth; // return x+strWidth; generates larger code 00106 // fall thru 00107 noString: 00108 return x; 00109 }
|
|
Draws a string at input coordinates using an alignment. Draw a string at x,y with alignment and return the new text position. Alignment determines which way the text is drawn. If you pass an alignment of Left:
If you pass an alignment of Right
If you pass an alignment of Center:
Definition at line 59 of file MoreWindow.c. 00060 { 00061 return MWinDrawAlignChars( str, MStrLen( str ), x, y, align ); 00062 }
|
|
Draws a string at input coordinates using an alignment, truncated to a maximum width. Draw a string at x,y with alignment and return the new text position, using a maximum of availWidth pixels. Alignment determines which way the text is drawn. If you pass an alignment of Left:
If you pass an alignment of Right
If you pass an alignment of Center:
Definition at line 84 of file MoreWindow.c. 00086 { 00087 return MWinDrawAlignCharsTrunc( str, MStrLen( str ), x, y, availWidth, 00088 align ); 00089 }
|
|
Switch screen back to default. MWinScreenToDefault switches the screen back to the default display mode. Definition at line 28 of file MoreWindow.c. 00029 {
00030 return WinScreenMode( winScreenModeSetToDefaults,
00031 NULL, NULL, NULL, NULL );
00032 }
|
|
Switch screen to a particular depth. MWinScreenToDepth switches the screen to a particular bit depth if available. If the depth is not available, sysErrParamErr is returned. Definition at line 36 of file MoreWindow.c. 00037 { 00038 Err err; 00039 UInt32 supportedDepths; 00040 UInt32 requiredDepth; 00041 // Get supported bit depths. 00042 err = WinScreenMode( winScreenModeGetSupportedDepths, NULL, NULL, 00043 &supportedDepths, NULL ); 00044 _reject( err, fail ) 00045 // Is the appropriate bit set? 00046 _require_action( ( supportedDepths & ( 1 << ( depth - 1 ) ) ), fail, 00047 err = sysErrParamErr; ); 00048 // If so, try to set to that depth. 00049 requiredDepth = depth; 00050 err = WinScreenMode( winScreenModeSet, NULL, NULL, &requiredDepth, NULL ); 00051 _reject( err, fail ); // dispatch notification in debug mode 00052 // err is errNone, so falling thru leads to right result 00053 fail: 00054 return err; 00055 }
|