MoreField.h File Reference
Functions for fields. More...
#include "MorePrefix.h"
#include "MoreTypes.h"
#include <Field.h>
#include <Form.h>
Include dependency graph for MoreField.h:
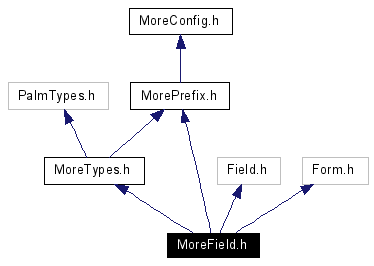
This graph shows which files directly or indirectly include this file:
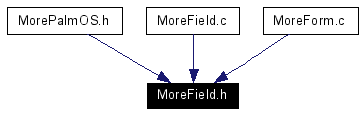
Go to the source code of this file.
Defines | |
#define | mstDefault 0 |
Typedefs | |
typedef UInt16 | MoreSetTextFlags |
Enumerations | |
enum | MoreSetTextFlag { mstDontRedraw = 0x0, mstRedraw = 0x1, mstReplaceOld = 0x0, mstReuseOld = 0x2, mstMemHandleFreeOld = 0x0, mstLeakOld = 0x4 } |
Functions | |
FieldType * | MFldGetByID (FormType *formP, FrmObjectID_t id) |
FieldType * | MFldGetByIdx (FormType *formP, FrmObjectIdx_t idx) |
FieldType * | MFldGetByPtr (FrmObject_t *objectP) |
Err | MFldSetTextFromChars (FieldType *fieldP, const char *s, UInt32 len, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromCharsByID (FormType *formP, FrmObjectID_t fieldID, const char *s, UInt32 len, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromCharsByIdx (FormType *formP, FrmObjectIdx_t fieldIdx, const char *s, UInt32 len, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromStr (FieldType *fieldP, const char *s, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromStrByID (FormType *formP, FrmObjectID_t fieldID, const char *s, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromStrByIdx (FormType *formP, FrmObjectIdx_t fieldIdx, const char *s, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldClearText (FieldType *fieldP, MoreSetTextFlags flags) |
Clears a field's text. | |
Err | MFldClearTextByID (FormType *formP, FrmObjectID_t fieldID, MoreSetTextFlags flags) |
Clears a field's text. | |
Err | MFldClearTextByIdx (FormType *formP, FrmObjectIdx_t fieldIdx, MoreSetTextFlags flags) |
Clears a field's text. | |
Err | MFldSetTextFromHandle (FieldPtr fieldP, MemHandle text, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromHandleByID (FormType *formP, FrmObjectID_t fieldID, MemHandle text, MoreSetTextFlags flags) |
Sets a field's text. | |
Err | MFldSetTextFromHandleByIdx (FormType *formP, FrmObjectIdx_t fieldIdx, MemHandle text, MoreSetTextFlags flags) |
Sets a field's text. |
Detailed Description
Functions for fields.
Definition in file MoreField.h.
Define Documentation
|
Definition at line 74 of file MoreField.h. |
Typedef Documentation
|
A MoreSetTextFlags controls the behaviour of the MoreFIeld set/clear functions. Options within each group are mutally exclusive, but you can combine one options from each group. Use the | operator to combine options or mstDefault for the default options. Redraw behaviour:
Old handle behaviour:
Disposal behaviour:
Definition at line 72 of file MoreField.h. |
Enumeration Type Documentation
|
Definition at line 75 of file MoreField.h. 00076 { 00077 mstDontRedraw = 0x0, 00078 mstRedraw = 0x1, 00079 00080 mstReplaceOld = 0x0, 00081 mstReuseOld = 0x2, 00082 00083 mstMemHandleFreeOld = 0x0, 00084 mstLeakOld = 0x4 00085 };
|
Function Documentation
|
Clears a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 175 of file MoreField.c. 00176 { 00177 _check( fieldP ); 00178 return MFldSetTextFromChars( fieldP, NULL, 0, flags ); 00179 }
|
|
Clears a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 181 of file MoreField.c. 00183 { 00184 _validate_object_id( formP, fieldID ); 00185 return MFldClearText( MFldGetByID( formP, fieldID ), flags ); 00186 }
|
|
Clears a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 188 of file MoreField.c. 00190 { 00191 _validate_object_idx( formP, fieldIdx ); 00192 return MFldClearText( MFldGetByIdx( formP, fieldIdx ), flags ); 00193 }
|
|
Definition at line 33 of file MoreField.c. 00034 { 00035 return ( FieldType* )MFrmGetObjPtrByID( formP, id ); 00036 }
|
|
Definition at line 38 of file MoreField.c. 00039 { 00040 return ( FieldType* )MFrmGetObjPtrByIdx( formP, idx ); 00041 }
|
|
Definition at line 43 of file MoreField.c. 00044 {
00045 return ( FieldType* )( objectP );
00046 }
|
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 74 of file MoreField.c. 00076 { 00077 Err err; 00078 UInt32 size = len + 1; 00079 MemHandle oldH = FldGetTextHandle( fieldP ); 00080 MemHandle newH = NULL; 00081 char* p; 00082 00083 // We've been asked to reuse the old hadnel. Try to do so. 00084 if ( ( mstReuseOld & flags ) && ( oldH ) ) 00085 { 00086 // Sever handle from field before changing it. 00087 FldSetTextHandle( fieldP, NULL ); 00088 err = MemHandleResize( oldH, size ); 00089 if ( errNone != err ) 00090 { 00091 /* Couldn't resize handle. Reattach it; we'll create a new 00092 one instead. */ 00093 FldSetTextHandle( fieldP, oldH ); 00094 } 00095 else 00096 { 00097 newH = oldH; 00098 } 00099 } 00100 00101 // Try to allocate a new handle for the text. 00102 if ( !newH ) 00103 { 00104 newH = MemHandleNew( size ); 00105 } 00106 00107 // If we still don't have a handle, fail. 00108 if ( !newH ) 00109 { 00110 return memErrNotEnoughSpace; 00111 } 00112 00113 // Copy stirng into handle. 00114 p = ( char * ) MemHandleLock( newH ); 00115 MemMove( p, s, len ); 00116 p [ len ] = 0; 00117 MemHandleUnlock( newH ); 00118 00119 /* Dispose of the old handle. We do this after copying in case the old 00120 handle contains the text we're copying. */ 00121 if ( oldH != newH ) 00122 PrvMFldAbandonHandle( oldH, flags ); 00123 00124 // Assign handle to field. 00125 FldSetTextHandle( fieldP, newH ); 00126 00127 // Redraw the field if so asked. 00128 PrvMFldRedraw( fieldP, flags ); 00129 00130 return errNone; 00131 }
|
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. |
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. |
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 196 of file MoreField.c. 00198 { 00199 Err err; 00200 if ( mstReuseOld & flags ) 00201 { 00202 /* We were asked to reuse the old handle, so lock down this handle 00203 and pass the text to MFldSetTextFromChars. */ 00204 MemPtr textP; 00205 UInt16 textSize; 00206 _validate_field_ptr( fieldP ); 00207 _validate_memhandle( text ); 00208 textSize = MemHandleSize( text ); 00209 textP = MemHandleLock( text ); 00210 err = MFldSetTextFromChars( fieldP, (char*)textP, textSize, flags ); 00211 /* Whether or not MFldSetTextFromChars() fails, we still need to 00212 unlock the text handle. */ 00213 MemHandleUnlock( text ); 00214 return err; 00215 } 00216 else 00217 { 00218 /* We weren't asked to reuse the old handle, so assign the new handle 00219 to the field. */ 00220 MemHandle oldH = FldGetTextHandle( fieldP ); 00221 if ( oldH != text ) /* Is this actually a new handle? */ 00222 { 00223 FldSetTextHandle( fieldP, text ); 00224 PrvMFldAbandonHandle( oldH, flags ); 00225 // Redraw the field if so asked. 00226 PrvMFldRedraw( fieldP, flags ); 00227 } 00228 return errNone; 00229 } 00230 }
|
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 232 of file MoreField.c. 00234 { 00235 _validate_object_id( formP, fieldID ); 00236 return MFldSetTextFromHandle( MFldGetByID( formP, fieldID ), text, 00237 flags ); 00238 }
|
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 240 of file MoreField.c. 00242 { 00243 _validate_object_idx( formP, fieldIdx ); 00244 return MFldSetTextFromHandle( MFldGetByIdx( formP, fieldIdx ), text, 00245 flags ); 00246 }
|
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. Definition at line 151 of file MoreField.c. 00153 { 00154 return MFldSetTextFromChars( fieldP, s, MStrLen( s ), flags ); 00155 }
|
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. |
|
Sets a field's text. Behaviour is determined by MoreSetTextFlags. |