MoreRect.h File Reference
Functions for points and rectangles. More...
#include "MorePrefix.h"
#include <Rect.h>
Include dependency graph for MoreRect.h:
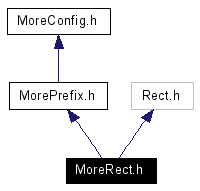
This graph shows which files directly or indirectly include this file:
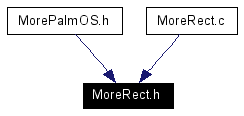
Go to the source code of this file.
Functions | |
void | MPtAddPoints (const PointType *point1P, const PointType *point2P, PointType *point3P) |
Adds two points. | |
void | MPtAddPoint (PointType *point1P, const PointType *point2P) |
Increments one point by another. | |
void | MPtSubtractPoint (PointType *point1P, const PointType *point2P) |
Decrements one point by another. | |
void | MRctPtsToRect (const PointType *point1P, const PointType *point2P, RectangleType *resultRectP) |
Calculates the smallest rectangle that contains two points. | |
void | MRctGetBottomRight (const RectangleType *rectP, PointType *bottomRight) |
Calculate the bottom right of a rectangle. | |
Coord | MRctFindXCenter (const RectangleType *rect1P) |
Calculates the horizontal center of a rectangle. | |
Coord | MRctFindYCenter (const RectangleType *rect1P) |
Calculates the vertical center of a rectangle. | |
Coord | MRctFindXCenterOf2 (const RectangleType *rect1P, const RectangleType *rect2P) |
Calculates the horizontal center of two rectangles. | |
Coord | MRctFindYCenterOf2 (const RectangleType *rect1P, const RectangleType *rect2P) |
Calculates the vertical center of two rectangles. | |
void | MRctGetUnion (const RectangleType *rect1P, const RectangleType *rect2P, RectangleType *r3P) |
Calculates the smallest rectangle that entirely contains two rectangles. | |
void | MRctRectToAbsRect (const RectangleType *rectP, AbsRectType *absRectP) |
Converts a RectangleType to an AbsRectType. | |
void | MRctAbsRectToRect (const AbsRectType *absRectP, RectangleType *rectP) |
Converts an AbsRectType to a RectangleType. |
Detailed Description
Functions for points and rectangles.
Definition in file MoreRect.h.
Function Documentation
|
Increments one point by another.
Definition at line 31 of file MoreRect.c. 00032 { 00033 point1P->x += point2P->x; 00034 point1P->y += point2P->y; 00035 }
|
|
Adds two points.
Definition at line 23 of file MoreRect.c. 00025 { 00026 *point3P = *point1P; 00027 point3P->x += point2P->x; 00028 point3P->y += point2P->y; 00029 }
|
|
Decrements one point by another.
Definition at line 37 of file MoreRect.c. 00038 { 00039 point1P->x -= point2P->x; 00040 point1P->y -= point2P->y; 00041 }
|
|
Converts an AbsRectType to a RectangleType. This function assumes the source retangle's coordinates are normalized (i.e. the top must be greather than the bottom, and the right must be greater than the left). Definition at line 130 of file MoreRect.c. 00131 { 00132 rectP->topLeft.x = absRectP->left; 00133 rectP->topLeft.y = absRectP->top; 00134 rectP->extent.x = absRectP->right - absRectP->left; 00135 rectP->extent.y = absRectP->bottom - absRectP->top; 00136 }
|
|
Calculates the horizontal center of a rectangle.
Definition at line 83 of file MoreRect.c. 00084 {
00085 return rectP->topLeft.x + (rectP->extent.x >> 1);
00086 }
|
|
Calculates the horizontal center of two rectangles.
Definition at line 93 of file MoreRect.c. 00095 { 00096 RectangleType rect3; 00097 MRctGetUnion( rect1P, rect2P, &rect3 ); 00098 return rect3.topLeft.x + (rect3.extent.x >> 1); 00099 }
|
|
Calculates the vertical center of a rectangle.
Definition at line 88 of file MoreRect.c. 00089 {
00090 return rectP->topLeft.y + (rectP->extent.y >> 1);
00091 }
|
|
Calculates the vertical center of two rectangles.
Definition at line 101 of file MoreRect.c. 00103 { 00104 RectangleType rect3; 00105 MRctGetUnion( rect1P, rect2P, &rect3 ); 00106 return rect3.topLeft.y + (rect3.extent.y >> 1); 00107 }
|
|
Calculate the bottom right of a rectangle.
Definition at line 77 of file MoreRect.c. 00078 { 00079 *bottomRight = rectP->topLeft; 00080 MPtAddPoint( bottomRight, &rectP->extent ); 00081 }
|
|
Calculates the smallest rectangle that entirely contains two rectangles.
Definition at line 109 of file MoreRect.c. 00111 { 00112 AbsRectType absRect1, absRect2, absRect3; 00113 MRctRectToAbsRect( rect1P, &absRect1 ); 00114 MRctRectToAbsRect( rect2P, &absRect2 ); 00115 absRect3.left = _min( absRect1.left, absRect2.left ); 00116 absRect3.top = _min( absRect1.top, absRect2.top ); 00117 absRect3.right = _max( absRect1.right, absRect2.right ); 00118 absRect3.bottom = _max( absRect1.bottom, absRect2.bottom ); 00119 MRctAbsRectToRect( &absRect3, rect3P ); 00120 }
|
|
Calculates the smallest rectangle that contains two points. This function does NOT assume any particular relationship between the two points. Definition at line 43 of file MoreRect.c. 00045 { 00046 Coord leftMost; 00047 Coord rightMost; 00048 Coord topMost; 00049 Coord bottomMost; 00050 if ( point1P->x < point2P->x ) 00051 { 00052 leftMost = point1P->x; 00053 rightMost = point2P->x; 00054 } 00055 else 00056 { 00057 leftMost = point2P->x; 00058 rightMost = point1P->x; 00059 } 00060 if ( point1P->y < point2P->y ) 00061 { 00062 topMost = point1P->y; 00063 bottomMost = point2P->y; 00064 } 00065 else 00066 { 00067 topMost = point1P->y; 00068 bottomMost = point1P->y; 00069 } 00070 resultRectP->topLeft.x = leftMost; 00071 resultRectP->topLeft.y = topMost; 00072 resultRectP->extent.x = rightMost - leftMost; 00073 resultRectP->extent.y = bottomMost - topMost; 00074 }
|
|
Converts a RectangleType to an AbsRectType.
Definition at line 122 of file MoreRect.c. 00123 { 00124 absRectP->left = rectP->topLeft.x; 00125 absRectP->top = rectP->topLeft.y; 00126 absRectP->right = rectP->topLeft.x + rectP->extent.x; 00127 absRectP->bottom = rectP->topLeft.y + rectP->extent.y; 00128 }
|