MoreControl.c File Reference
#include "MorePrefix.h"
#include "MoreControl.h"
#include "MoreForm.h"
#include "MorePrivate.h"
Include dependency graph for MoreControl.c:
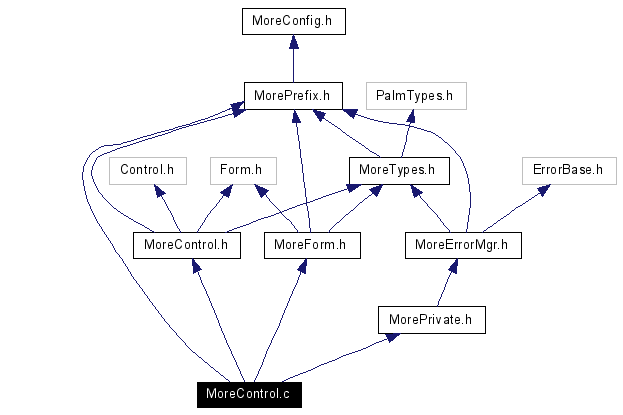
Go to the source code of this file.
Functions | |
ControlType * | MCtlGetPtrByIdx (const FormType *formP, FrmObjectIdx_t idx) |
Returns a control given its form and object index. | |
ControlType * | MCtlGetPtrByID (const FormType *formP, FrmObjectID_t id) |
Returns a control given its form and object id. | |
ControlType * | MCtlGetPtrByObjPtr (FrmObject_t *objectP) |
Returns a control given its object pointer. | |
ControlType * | MCtlGetPtrByIdxSafely (const FormType *formP, FrmObjectIdx_t idx) |
Returns a control given its form and object index. | |
ControlType * | MCtlGetPtrByIDSafely (const FormType *formP, FrmObjectID_t id) |
Returns a control given its form and object id. | |
ControlType * | MCtlGetPtrByObjPtrSafely (const FormType *formP, FrmObject_t *objectP) |
Returns a control given its object pointer. | |
Boolean | MCtlGetEnabledByID (const FormType *formP, FrmObjectID_t id) |
Returns if a control is enabled. | |
Boolean | MCtlGetEnabledByIdx (const FormType *formP, FrmObjectIdx_t idx) |
Returns if a control is enabled. | |
Boolean | MCtlGetEnabledByPtr (ControlType *controlP) |
Returns if a control is enabled. | |
void | MCtlSetEnabledByID (const FormType *formP, FrmObjectID_t id, Boolean enabled) |
Sets wwhether a control is enabled. | |
void | MCtlSetEnabledByIdx (const FormType *formP, FrmObjectIdx_t idx, Boolean enabled) |
Sets wwhether a control is enabled. | |
void | MCtlSetEnabled (ControlType *controlP, Boolean enabled) |
Sets wwhether a control is enabled. | |
CtlValue_t | MCtlGetValueByID (const FormType *formP, FrmObjectID_t id) |
Returns a control's value. | |
CtlValue_t | MCtlGetValueByIdx (const FormType *formP, FrmObjectIdx_t idx) |
Returns a control's value. | |
CtlValue_t | MCtlGetValue (ControlType *controlP) |
Returns a control's value. | |
void | MCtlSetValueByID (FormType *formP, FrmObjectID_t id, CtlValue_t value) |
Sets a control's value. | |
void | MCtlSetValueByIdx (FormType *formP, FrmObjectIdx_t idx, CtlValue_t value) |
Sets a control's value. | |
void | MCtlSetValue (ControlType *ctrlP, CtlValue_t value) |
Sets a control's value. | |
void | MCtlCopyValueByID (FormType *formP, FrmObjectID_t srcID, FrmObjectID_t dstID) |
Copies one control's value to another. | |
void | MCtlCopyValueByIdx (FormType *formP, FrmObjectIdx_t srcIdx, FrmObjectIdx_t dstIdx) |
Copies one control's value to another. | |
void | MCtlCopyValue (ControlType *srcCtrlP, ControlType *dstCtrlP) |
Copies one control's value to another. |
Function Documentation
|
Copies one control's value to another. Given a form and two control pointers, this function sets one control's value to the value of the other. If the destination control is visible, it's redrawn. Preconditions:
Definition at line 138 of file MoreControl.c. 00139 { 00140 CtlSetValue( dstCtrlP, CtlGetValue( srcCtrlP ) ); 00141 }
|
|
Copies one control's value to another. Given a form and two object ids, this function sets one control's value to the value of the other. If the destination control is visible, it's redrawn. Preconditions:
Definition at line 128 of file MoreControl.c. 00129 { 00130 MCtlSetValueByID( formP, dstID, MCtlGetValueByID( formP, srcID ) ); 00131 }
|
|
Copies one control's value to another. Given a form and two object indexes, this function sets one control's value to the value of the other. If the destionation control is visible, it's redrawn. Preconditions:
Definition at line 133 of file MoreControl.c. 00134 { 00135 MCtlSetValueByIdx( formP, dstIdx, MCtlGetValueByID( formP, srcIdx ) ); 00136 }
|
|
Returns if a control is enabled. Given a form and object id, this function returns whether the control is enabled. Preconditions:
Definition at line 60 of file MoreControl.c. 00061 { 00062 return CtlEnabled( MCtlGetPtrByID( formP, id ) ); 00063 }
|
|
Returns if a control is enabled. Given a form and object index, this function returns whether the control is enabled. Preconditions:
Definition at line 65 of file MoreControl.c. 00066 { 00067 return CtlEnabled( MCtlGetPtrByIdx( formP, idx ) ); 00068 }
|
|
Returns if a control is enabled. Given a control pointer, this function returns whether the control is enabled. Preconditions:
Definition at line 70 of file MoreControl.c. 00071 {
00072 return CtlEnabled( controlP );
00073 }
|
|
Returns a control given its form and object id. Given a form and object id, this function returns the corresponding control. This is just like type casting MFrmGetObjPtrByID. Preconditions:
Definition at line 31 of file MoreControl.c. 00032 { 00033 return ( ControlType* ) MFrmGetObjPtrByID( formP, id ); 00034 }
|
|
Returns a control given its form and object id. Given a form and object id, this function returns the corresponding control. This is just like type casting MFrmGetObjPtrByID, but a safe type cast is done for you (i.e. if the object exists but is not a control, NULL will be returned). Preconditions:
Definition at line 48 of file MoreControl.c. 00049 { 00050 return ( ControlType* ) MFrmGetObjPtrOfTypeByID( formP, id, frmControlObj ); 00051 }
|
|
Returns a control given its form and object index. Given a form and object index, this function returns the corresponding control. This is just like type casting MFrmGetObjPtrByIdx. Preconditions:
Definition at line 26 of file MoreControl.c. 00027 { 00028 return ( ControlType* ) MFrmGetObjPtrByIdx( formP, idx ); 00029 }
|
|
Returns a control given its form and object index. Given a form and object index, this function returns the corresponding control. This is just like type casting MFrmGetObjPtrByIdx, but a safe type cast is done for you (i.e. if the object exists but is not a control, NULL will be returned). Preconditions:
Definition at line 43 of file MoreControl.c. 00044 { 00045 return ( ControlType* ) MFrmGetObjPtrOfTypeByIdx( formP, idx, frmControlObj ); 00046 }
|
|
Returns a control given its object pointer. Given an object pointer, this function returns the corresponding control. This is just like type casting MFrmGetObjPtrByID. Preconditions:
Definition at line 36 of file MoreControl.c. 00037 {
00038 return ( ControlType* ) ( objectP );
00039 }
|
|
Returns a control given its object pointer. Given an object pointer, this function returns the corresponding control. This is just like type casting MFrmGetObjPtrByID, but a safe tpe cast is done for you (i.e. if the object exists but is not a control, NULL will be returned). Preconditions:
Definition at line 53 of file MoreControl.c. 00054 { 00055 return ( ControlType* ) MFrmGetObjPtrOfTypeByPtr( formP, objectP, frmControlObj ); 00056 }
|
|
Returns a control's value. Given a form and object id, this function returns the control's value. If the control is visible, it's redrawn. Preconditions:
Definition at line 104 of file MoreControl.c. 00105 {
00106 return CtlGetValue( controlP );
00107 }
|
|
Returns a control's value. Given a form and object id, this function returns the control's value. Preconditions:
Definition at line 94 of file MoreControl.c. 00095 { 00096 return CtlGetValue( MCtlGetPtrByID( formP, id ) ); 00097 }
|
|
Returns a control's value. Given a form and object index, this function returns the control's value. Preconditions:
Definition at line 99 of file MoreControl.c. 00100 { 00101 return CtlGetValue( MCtlGetPtrByID( formP, idx ) ); 00102 }
|
|
Sets wwhether a control is enabled. Given a control pointer, this function sets whether the control is enabled. Preconditions:
Definition at line 87 of file MoreControl.c. 00088 { 00089 CtlSetEnabled( controlP, enabled ); 00090 }
|
|
Sets wwhether a control is enabled. Given a form and object id, this function sets whether the control is enabled. Preconditions:
Definition at line 77 of file MoreControl.c. 00078 { 00079 CtlSetEnabled( MCtlGetPtrByID( formP, id ), enabled ); 00080 }
|
|
Sets wwhether a control is enabled. Given a form and object index, this function sets whether the control is enabled. Preconditions:
Definition at line 82 of file MoreControl.c. 00083 { 00084 CtlSetEnabled( MCtlGetPtrByIdx( formP, idx ), enabled ); 00085 }
|
|
Sets a control's value. Given a form and object id, this function sets the control's value. If the control is visible, it's redrawn. Preconditions:
Definition at line 121 of file MoreControl.c. 00122 { 00123 CtlSetValue( ctrlP, value ); 00124 }
|
|
Sets a control's value. Given a form and object id, this function sets the control's value. If the control is visible, it's redrawn. Preconditions:
Definition at line 111 of file MoreControl.c. 00112 { 00113 CtlSetValue( MCtlGetPtrByID( formP, id ), value ); 00114 }
|
|
Sets a control's value. Given a form and object index, this function sets the control's value. Preconditions:
Definition at line 116 of file MoreControl.c. 00117 { 00118 CtlSetValue( MCtlGetPtrByID( formP, idx ), value ); 00119 }
|